Basic Shapes
IPKISS shapes are all subclasses of the basic Shape
class.
Basic shape |
|
Shape defined by a parametric function |
|
Basic circle |
|
Circular arc |
|
Circular arc specified by its starting point instead of its center |
|
Bend with relative turning angle instead of absolute end angle |
|
Cross. |
|
Wedge, or symmetric trapezium. |
|
Radial wedge: the coordinates of the start and end point are specified in polar coordinates from a given center |
|
Basic ellipse |
|
Ellipse arc around a given center. |
|
Basic rectangle |
|
Rectangle with rounded corners |
|
Ring segment |
|
Regular N-sided polygon |
|
Hexagon |
|
Dodecagon |
|
Parabolic wedge (taper) |
|
Exponential wedge (taper) |
|
Raised Sine S-bend |
|
Cosine S-bend |
|
Radial S-bend |
Shape
- class ipkiss3.all.Shape
Basic shape
- Parameters:
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
>>> shape = Shape(points=[(0.0, 0.0), (10.0, 10.0)]) >>> shape.points # returns the points of the shape >>> array([[ 0., 0.], >>> [ 10., 10.]])
>>> shape = Shape(points=[(0.0, 0.0), (10.0, 10.0)]) >>> len(shape) # returns the number of points of the shape >>> 2
>>> shape.get_face_angles() # returns (start_face_angle, end_face_angle), >>> (45.0, 45.0) # these are the angles at the start and the end of an open shape.
>>> shape.closed # returns whether the shape is closed or not. >>> False # If you set closed=True, the shape will be filled.
>>> wg_layout = i3.Waveguide().Layout(shape=shape) >>> wg_layout.visualize()
The start_face_angle is not equivalent to the waveguide port angle, as it points inwards and not outwards. The end_face_angle, however, is equivalent to the waveguide port angle.
>>> shape.start_face_angle = 10. >>> wg_layout2 = i3.Waveguide().Layout(shape=shape) >>> wg_layout2.visualize()
Examples
import ipkiss3.all as i3
import matplotlib.pyplot as plt
s1 = i3.Shape([(5.0, -5.0), (0.0, 0.0), (5.0, 5.0), (10.0, 0.0)], closed=False)
p2 = i3.Coord2((15.0, 0.0))
s2 = s1 + p2
plt.figure()
plt.plot(s2.x_coords(), s2.y_coords(), 'bo-', markersize=8, linewidth=2)
plt.show()
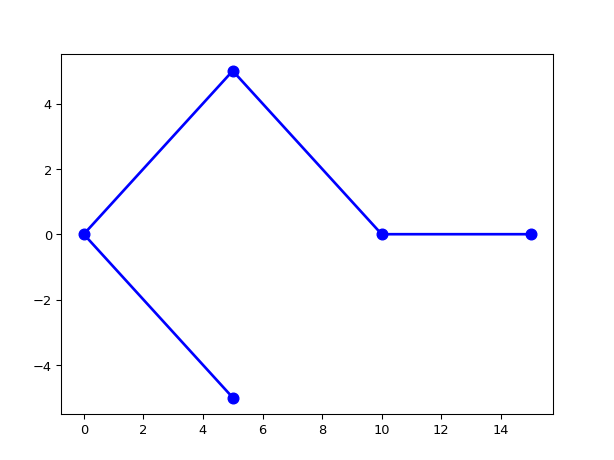
ParametricShape
- class ipkiss3.all.ParametricShape
Shape defined by a parametric function
The shape is defined by a function curve(t) -> x(t), y(t) The normalized parameter t is a floating point value varied between 0.0 and t_max to generate the curve.
For instance, the equations x = cos(t) and y = sin(t) form the parametric equation of the unit circle. Sensible values for t depend on the equations. The property t_max can be set to choose the maximum t value the curve function is evaluated for.
A classical iterative midpoint sampling algorithm is used for calculating the shape. In each iteration, the list of t sample values is updated with the midpoints between each pair of t values, and is then evaluated to get the updated x,y coordinates.
Additional sample points will be added to the shape until adding a new sample point is deviating minimally from a straight line through its neighbours. The accuracy of the curve can be tweaked by choosing the maximum deviation (max_deviation property).
By default, the algorithm starts from the start and end of the curve, [0.0, t_max], as the sample points. Since for several shapes (e.g. a circle) this does not give the desired outcome, the initial sample points can be chosen by setting initial_t. This should be a monotonically increasing list starting with 0.0 and ending with t_max (or monotonically decreasing if t_max is negative).
The algorithm stops hard after max_refine_depth iterations even if the desired accuracy max_deviation is not reached yet, in order to avoid too deep iteration.
- Parameters:
- max_refine_depth: int, optional
maximum number of refinement iterations
- initial_t: list, optional
monotonically increasing list of initial values between 0 and t_max, for which the curve function is evaluated to bootstrap the curve.
- t_max: float, optional
maximum value of the parameter t, defaults to 1.0
- max_deviation: float, optional
maximum deviation of the discretized points from the analytical curve
- curve: optional
Parametric curve function curve(t) returning x and y for the given t
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
"""Half ellipse defined as parametric curve""" import numpy as np from typing import Tuple import ipkiss3.all as i3 import matplotlib.pyplot as plt from matplotlib.patches import Polygon def curve(t: float) -> Tuple[float, float]: x = 10 * np.cos(t) y = 5 * np.sin(t) return x, y fig, ax = plt.subplots() shape = i3.ParametricShape(curve=curve, initial_t=np.linspace(0, np.pi, 50).tolist(), t_max=np.pi) SI = shape.size_info margin = 0.5 ax.set_xlim((SI.west - margin, SI.east + margin)) ax.set_ylim((SI.south - margin, SI.north + margin)) ax.grid(True) ax.axis("equal") ax.add_patch(Polygon(shape.points, closed=shape.is_closed())) plt.show()
"""Euler spiral defined as parametric curve""" from typing import Tuple import ipkiss3.all as i3 import pylab as plt from scipy.special import fresnel # fresnel(2) is the first full loop, calculate the scaling to have the first loop pass at a given x coordinate distance_x = 5.0 scaling = distance_x / fresnel(2)[0] def curve(t: float) -> Tuple[float, float]: x, y = fresnel(t) return scaling * x, scaling * y shape = i3.ParametricShape(curve=curve, t_max=2.5) fig, ax = plt.subplots() ax.plot(shape.x_coords(), shape.y_coords(), "o-") ax.set_title("Parametric Euler spiral [0.0-2.5]") ax.set_xlabel("x [um]") ax.set_ylabel("y [um]") ax.set_aspect("equal") plt.show()
ShapeCircle
- class ipkiss3.all.ShapeCircle
Basic circle
- Parameters:
- radius: float and number > 0, optional
radius of the circular arc
- clockwise: ( bool, bool_ or int ), optional
orientation of the arc. clockwise:True
- angle_step: float, optional
discretization angle
- end_angle: optional
- start_angle: optional
- box_size: optional
- center: Coord2, optional
center of the ellipse
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeCircle(radius=2.0))
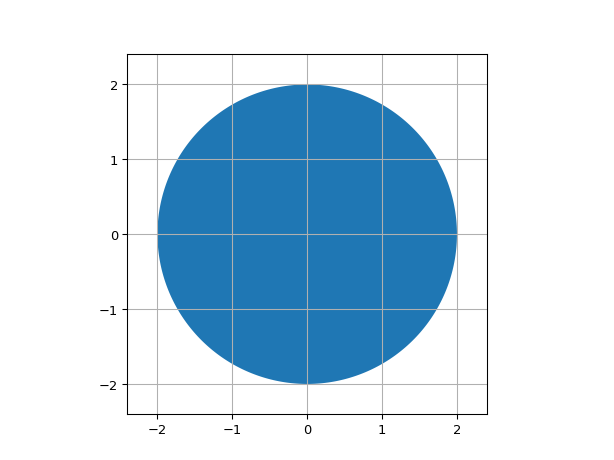
ShapeArc
- class ipkiss3.all.ShapeArc
Circular arc
- Parameters:
- radius: float and number > 0, optional
radius of the circular arc
- clockwise: ( bool, bool_ or int ), optional
orientation of the arc. clockwise:True
- angle_step: float, optional
discretization angle
- end_angle: float, optional
end angle of the arc according to the parametric representation of an ellipse
- start_angle: float, optional
start angle of the arc according to the parametric representation of an ellipse
- box_size: optional
- center: Coord2, optional
center of the ellipse
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeArc(start_angle=0,
end_angle=90.0 + 45.0))
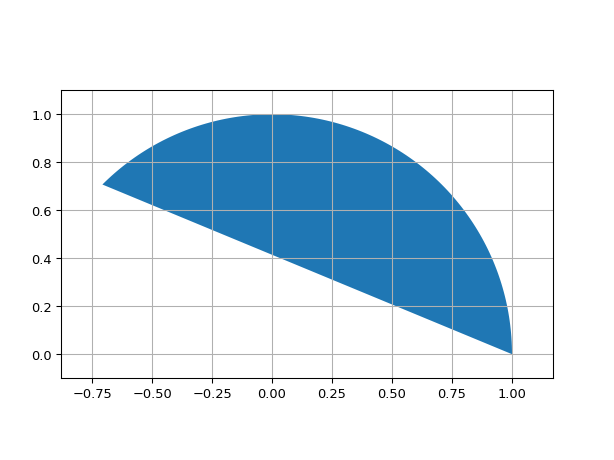
ShapeBend
- class ipkiss3.all.ShapeBend
Circular arc specified by its starting point instead of its center
- Parameters:
- output_angle: float, optional
- input_angle: float, optional
- start_point: Coord2, optional
starting point of the circular bend
- radius: float and number > 0, optional
radius of the circular arc
- clockwise: ( bool, bool_ or int ), optional
orientation of the arc. clockwise:True
- angle_step: float, optional
discretization angle
- end_angle: optional
end angle in degrees
- start_angle: optional
start angle in degrees
- box_size: optional
- center: optional
center of the bend
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeBend(start_point=(0.0, -1.0), start_angle=270.0, end_angle=310.0))
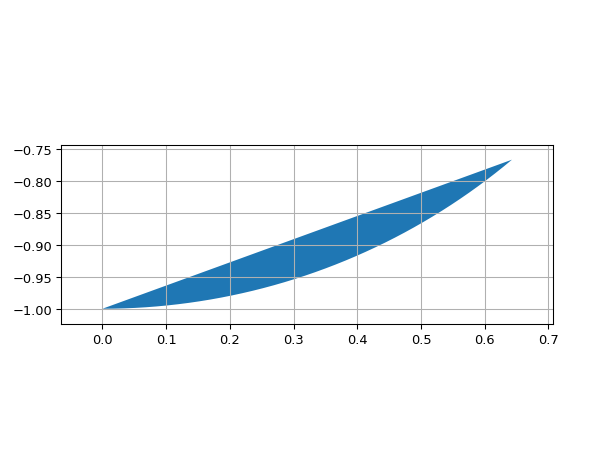
ShapeBendRelative
- ipkiss3.all.ShapeBendRelative(start_point=(0.0, 0.0), radius=1.0, input_angle=0.0, angle_amount=90.0, angle_step=1.0, **kwargs)
Bend with relative turning angle instead of absolute end angle
Examples
import ipkiss3.all as i3
import pylab as plt
# You can set the start_point to move the bend shapes, such as ShapeBend, ShapeBendRelative and S-bend Shapes.
shape = i3.ShapeBendRelative(start_point=(0.0, -1.0), input_angle=90.0, angle_amount=65.0)
plt.plot(shape.x_coords(), shape.y_coords(), 'bo-')
plt.gca().set_aspect('equal')
plt.show()
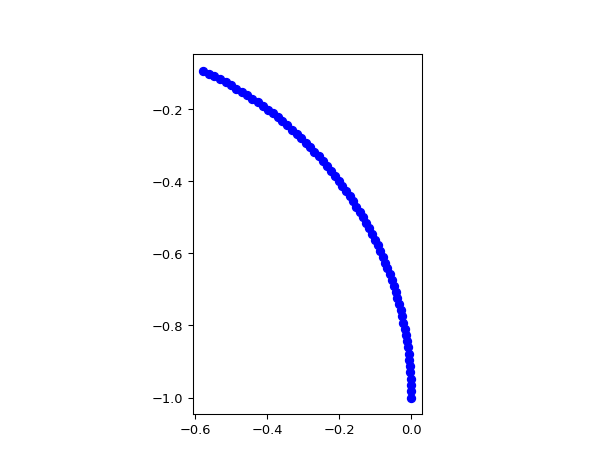
ShapeCross
- class ipkiss3.all.ShapeCross
Cross. Thickness sets the width of the arms
- Parameters:
- thickness: float and number > 0, optional
- box_size: float and number > 0, optional
- center: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeCross(center=(5.0, 0.0),
thickness=1.0))
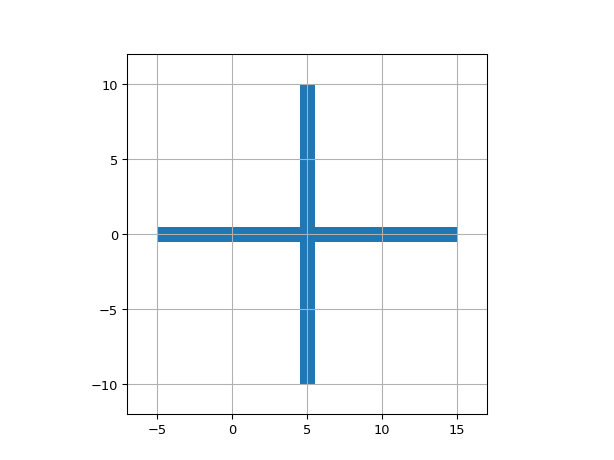
ShapeWedge
- class ipkiss3.all.ShapeWedge
Wedge, or symmetric trapezium. Specified by the center of baselines and the length of the baselines
- Parameters:
- end_width: float and Real, number and number >= 0, optional
- begin_width: float and Real, number and number >= 0, optional
- end_coord: Coord2, optional
- begin_coord: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeWedge(begin_coord=(0.0, 0.0), end_coord=(10.0, 0.0), begin_width=4.0, end_width=1.0))
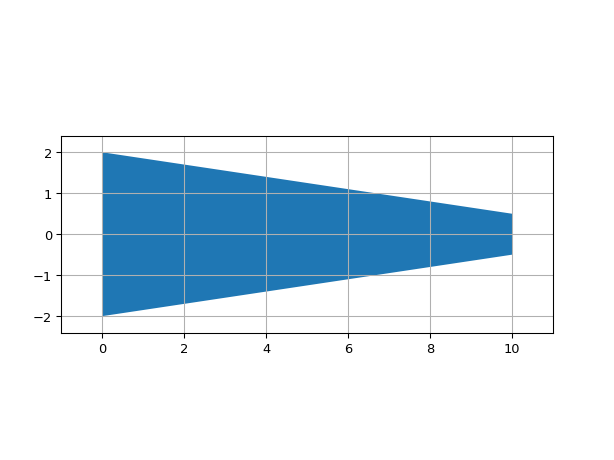
ShapeRadialWedge
- class ipkiss3.all.ShapeRadialWedge
Radial wedge: the coordinates of the start and end point are specified in polar coordinates from a given center
- Parameters:
- angle: float, required
- outer_width: float and number > 0, required
- inner_width: float and number > 0, required
- outer_radius: float and number > 0, required
- inner_radius: float and number > 0, required
- center: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeRadialWedge(inner_radius=5.0, outer_radius=15.0,
inner_width=1.0, outer_width=3.0,
angle=20.0))
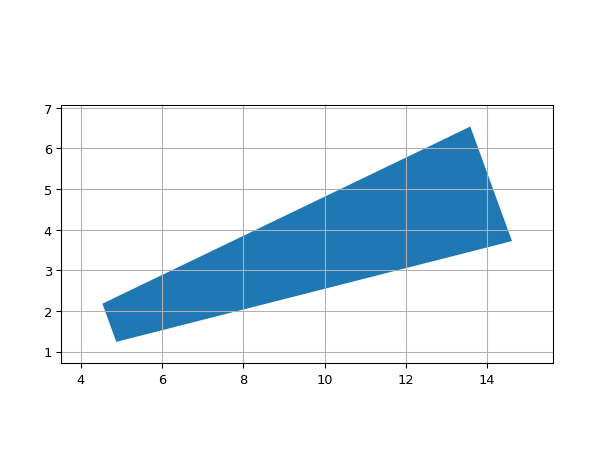
ShapeEllipse
- class ipkiss3.all.ShapeEllipse
Basic ellipse
- Parameters:
- clockwise: ( bool, bool_ or int ), optional
orientation of the arc. clockwise:True
- angle_step: float, optional
discretization angle
- end_angle: optional
- start_angle: optional
- box_size: Coord2 and number >= 0, optional
size of the ellipse along major and minor axis
- center: Coord2, optional
center of the ellipse
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeEllipse(start_angle=45.0,
end_angle=180.0))
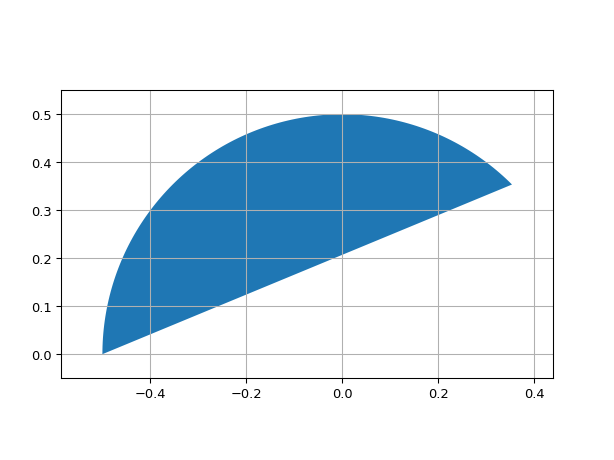
ShapeEllipseArc
- class ipkiss3.all.ShapeEllipseArc
Ellipse arc around a given center.
ShapeEllipseArc implements the standard parametric representation of an ellipse (x = a * cos(t), y = b * sin(t)) and (0 <= t < 2 * pi), where parameter t is not the actual angle, but has a geometric meaning due to Philippe de La Hire.
The start_angle and end_angle are described as this parameter t.
- Parameters:
- clockwise: ( bool, bool_ or int ), optional
orientation of the arc. clockwise:True
- angle_step: float, optional
discretization angle
- end_angle: float, optional
end angle of the arc according to the parametric representation of an ellipse
- start_angle: float, optional
start angle of the arc according to the parametric representation of an ellipse
- box_size: Coord2 and number >= 0, optional
size of the ellipse along major and minor axis
- center: Coord2, optional
center of the ellipse
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Notes
If you want to get the parametric angle t from the actual angle alpha, you can use the following:
import numpy as np a, b = box_size t = np.arctan2(2. / b * np.sin(alpha), 2. / a * np.cos(alpha)) # t and alpha in radians
Examples
import ipkiss.all as ia
visualize(ia.ShapeEllipseArc(start_angle=45.0,
center=(1.0, 1.0)))
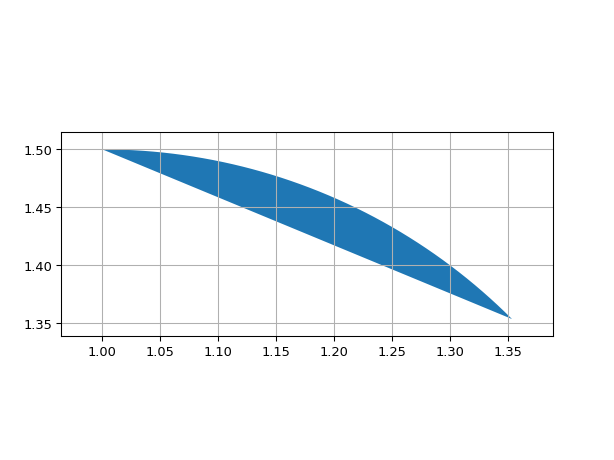
ShapeRectangle
- class ipkiss3.all.ShapeRectangle
Basic rectangle
- Parameters:
- angle_step: float, optional
angle_step using in the rounding.
- radius: optional
- box_size: Coord2 and number >= 0, optional
size of the rectangle.
- center: Coord2, optional
center of the rectangle.
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeRectangle(center=(1.5, 1.0),
box_size=(3.0, 2. )))
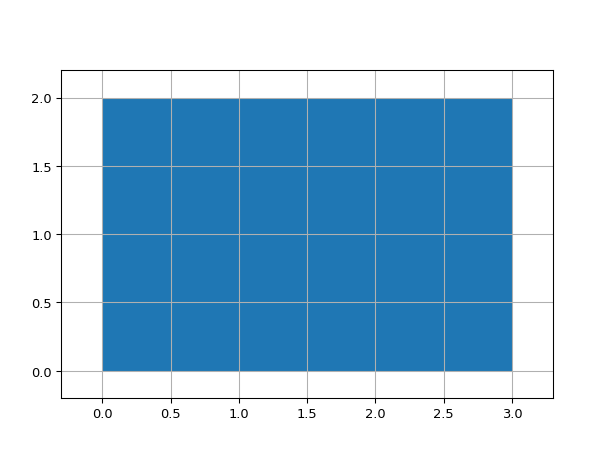
ShapeRoundedRectangle
- class ipkiss3.all.ShapeRoundedRectangle
Rectangle with rounded corners
- Parameters:
- angle_step: float, optional
angle_step using in the rounding.
- radius: float and Real, number and number >= 0, optional
radius of the rounding used.
- box_size: Coord2 and number >= 0, optional
size of the rectangle.
- center: Coord2, optional
center of the rectangle.
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeRoundedRectangle(center=(0.0, 0.0),
box_size=(1.0, 1.0),
radius=0.2))
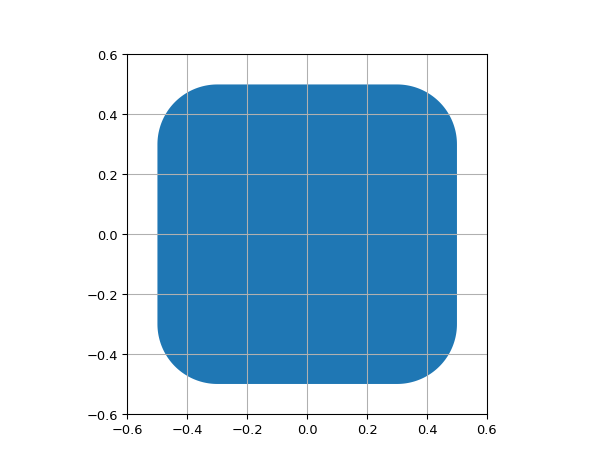
ShapeRingSegment
- class ipkiss3.all.ShapeRingSegment
Ring segment
- Parameters:
- outer_radius: float and number > 0, required
- inner_radius: float and number > 0, required
- angle_step: float, optional
- angle_end: float, optional
- angle_start: float, optional
- center: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeRingSegment(center=(0.0, 0.0),
angle_start=45.0,
angle_end=45.0 + 90.0,
inner_radius=9.0,
outer_radius=10.0))
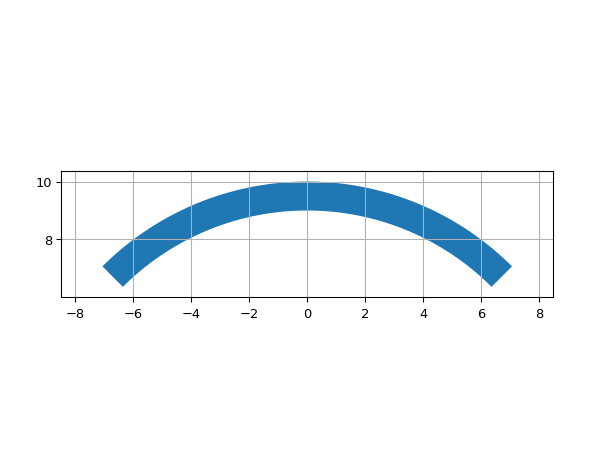
ShapeHexagon
- class ipkiss3.all.ShapeHexagon
Hexagon
- Parameters:
- radius: float and number > 0, optional
Radius of the polygon
- center: Coord2, optional
Center of the polygon
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- n_o_sides: int and [3,None], locked
Number of sides of the hexagon
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeHexagon(center=(0.0, 0.0),
radius=5.0))
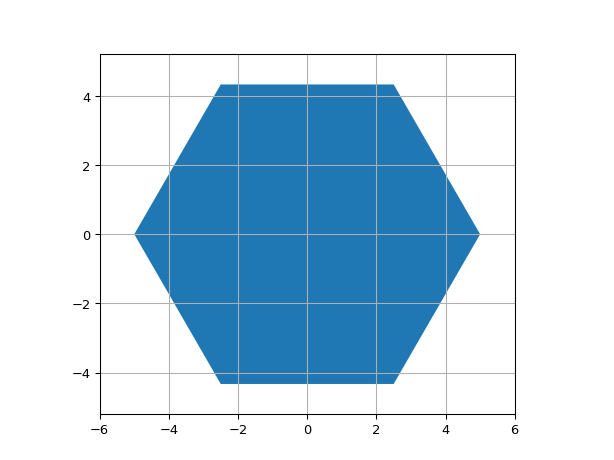
ShapeDodecagon
- class ipkiss3.all.ShapeDodecagon
Dodecagon
- Parameters:
- n_o_sides: int and [3,None], optional
- radius: float and number > 0, optional
Radius of the polygon
- center: Coord2, optional
Center of the polygon
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeDodecagon(center=(0.0, 0.0),
radius=5.0))
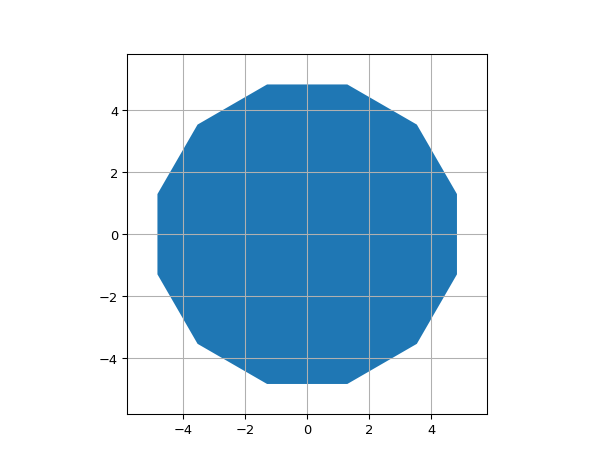
ShapeRegularPolygon
- class ipkiss3.all.ShapeRegularPolygon
Regular N-sided polygon
- Parameters:
- n_o_sides: int and [3,None], optional
Number of sides fo the polygon
- radius: float and number > 0, optional
Radius of the polygon
- center: Coord2, optional
Center of the polygon
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeRegularPolygon(center=(0.0, 0.0),
n_o_sides=12, radius=5.0))
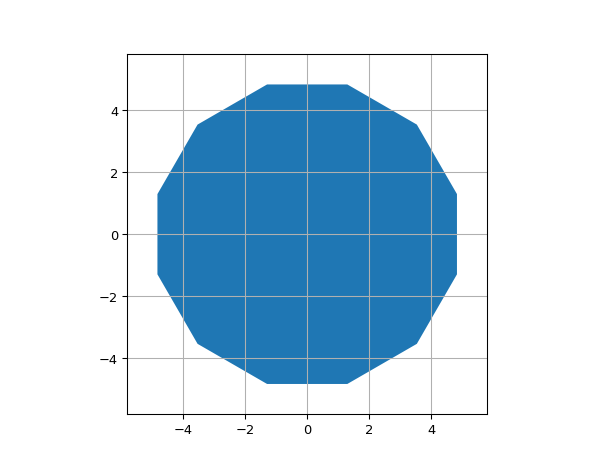
ShapeParabolic
- class ipkiss3.all.ShapeParabolic
Parabolic wedge (taper)
- Parameters:
- width_step: float and number > 0, optional
- end_width: float and Real, number and number >= 0, optional
- begin_width: float and Real, number and number >= 0, optional
- end_coord: Coord2, optional
- begin_coord: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeParabolic(begin_coord=(-2.0, 0.0),
end_coord=(2.0, 0.0),
begin_width=3.0,
end_width=1.0))
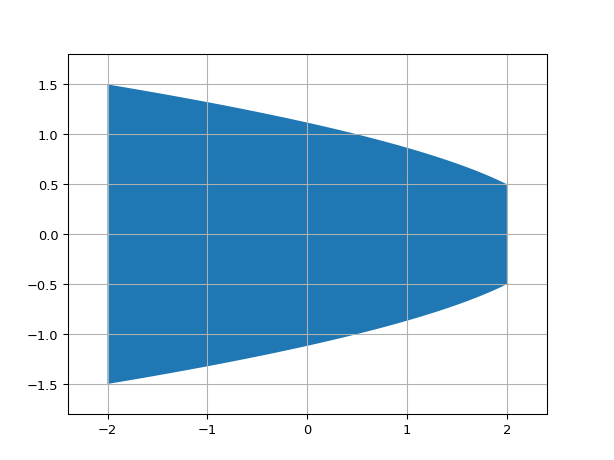
ShapeExponential
- class ipkiss3.all.ShapeExponential
Exponential wedge (taper)
- Parameters:
- g: float and Real, number and number >= 0, optional
Exponential growth constant. Defaults to ln(max_width/min_width)
- width_step: float and number > 0, optional
- end_width: float and Real, number and number >= 0, optional
- begin_width: float and Real, number and number >= 0, optional
- end_coord: Coord2, optional
- begin_coord: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss.all as ia
visualize(ia.ShapeExponential(begin_coord=(-2.0, 0.0),
end_coord=(2.0, 0.0),
begin_width=3.0,
end_width=1.0))
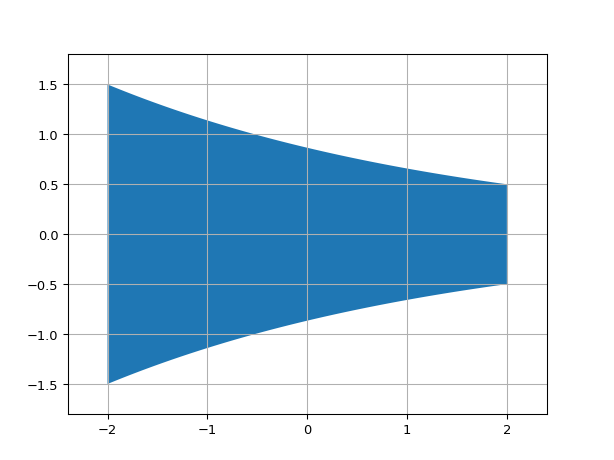
S-bend Shapes
- class ipkiss3.all.ShapeSineSBend
Raised Sine S-bend
- Parameters:
- y_offset: float, required
transversal offset the bend makes.
- x_offset: float, required
offset in x covered by the bend.
- start_angle: float, optional
start angle of the bend
- start_point: Coord2, optional
start point of the bend
- max_refine_depth: int, optional
maximum number of refinement iterations
- initial_t: list, optional
monotonically increasing list of initial values between 0 and t_max, for which the curve function is evaluated to bootstrap the curve.
- max_deviation: float, optional
maximum deviation of the discretized points from the analytical curve
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- t_max: float, locked
- curve: locked
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss3.all as i3 import pylab as plt shape_sine = i3.ShapeSineSBend(x_offset=30.0, y_offset=-20.0) fig, ax = plt.subplots() ax.plot(shape_sine.x_coords(), shape_sine.y_coords(), 'bo-') ax.set_xlabel('x [um]') ax.set_ylabel('y [um]') ax.set_title('Sine s-bend') ax.set_aspect('equal') plt.show()
- class ipkiss3.all.ShapeCosineSBend
Cosine S-bend
- Parameters:
- y_offset: float, required
transversal offset the bend makes.
- x_offset: float, required
offset in x covered by the bend.
- start_angle: float, optional
start angle of the bend
- start_point: Coord2, optional
start point of the bend
- max_refine_depth: int, optional
maximum number of refinement iterations
- initial_t: list, optional
monotonically increasing list of initial values between 0 and t_max, for which the curve function is evaluated to bootstrap the curve.
- max_deviation: float, optional
maximum deviation of the discretized points from the analytical curve
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- t_max: float, locked
- curve: locked
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss3.all as i3 import pylab as plt shape_cosine = i3.ShapeCosineSBend(x_offset=30.0, y_offset=-20.0) fig, ax = plt.subplots() ax.plot(shape_cosine.x_coords(), shape_cosine.y_coords(), 'bo-') ax.set_xlabel('x [um]') ax.set_ylabel('y [um]') ax.set_title('Cosine s-bend') ax.set_aspect('equal') plt.show()
- class ipkiss3.all.ShapeRadialSBend
Radial S-bend
- Parameters:
- y_offset: float, required
transversal offset the bend makes.
- x_offset: float, required
offset in x covered by the bend.
- start_angle: float, optional
start angle of the bend
- start_point: Coord2, optional
start point of the bend
- max_refine_depth: int, optional
maximum number of refinement iterations
- initial_t: list, optional
monotonically increasing list of initial values between 0 and t_max, for which the curve function is evaluated to bootstrap the curve.
- max_deviation: float, optional
maximum deviation of the discretized points from the analytical curve
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- points: optional
points of this shape
- Other Parameters:
- t_max: float, locked
- curve: locked
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
import ipkiss3.all as i3 import pylab as plt shape_radial = i3.ShapeRadialSBend(x_offset=30.0, y_offset=-20.0) fig, ax = plt.subplots() ax.plot(shape_radial.x_coords(), shape_radial.y_coords(), 'bo-') ax.set_xlabel('x [um]') ax.set_ylabel('y [um]') ax.set_title('Radial s-bend') ax.set_aspect('equal') plt.show()
Examples
import ipkiss3.all as i3
import pylab as plt
L = 50.0
H = 15.0
sh_sine = i3.ShapeSineSBend(x_offset=L, y_offset=H)
sh_cosine = i3.ShapeCosineSBend(x_offset=L, y_offset=H)
sh_radial = i3.ShapeRadialSBend(x_offset=L, y_offset=H)
plt.plot(sh_sine.x_coords(), sh_sine.y_coords(), 'bo-', label='sine')
plt.plot(sh_cosine.x_coords(), sh_cosine.y_coords(), 'ro-', label='cosine')
plt.plot(sh_radial.x_coords(), sh_radial.y_coords(), 'yo-', label='radial')
plt.xlabel('x [um]')
plt.ylabel('y [um]')
plt.legend()
plt.title('Sine, cosine and radial s-bend')
plt.show()
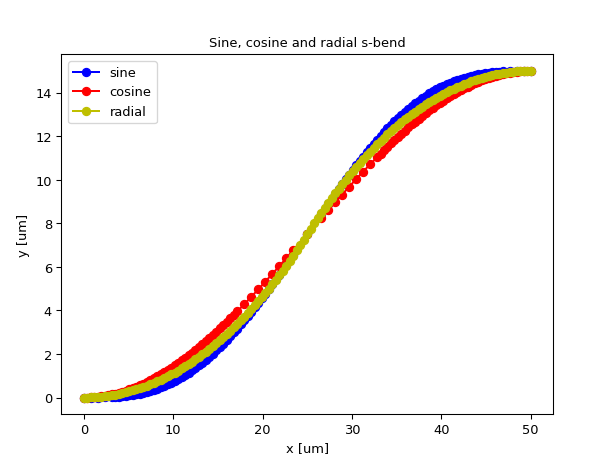